When pressing buttons on FPGA, there are unpredictable bounces that are unwanted. This VHDL code is to debounce buttons on FPGA by only generating a single pulse with a period of the input clock when the button on FPGA is pressed, held long enough, and released. Last time, I presented a simple Verilog code for debouncing buttons on FPGA.
This VHDL project is to present a VHDL code for debouncing buttons on FPGA. Full VHDL code and testbench are provided.
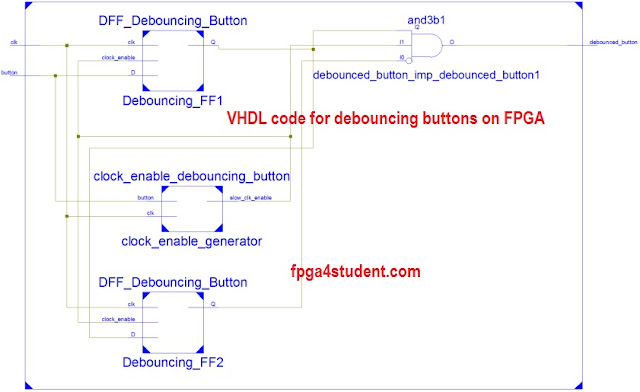
VHDL code for debouncing buttons on FPGA:
--fpga4student.com: FPGA projects, Verilog projects, VHDL projects -- VHDL project: VHDL code for debouncing buttons on FPGA -- Generate Slow clock enable for debouncing buttons library IEEE; use IEEE.STD_LOGIC_1164.ALL; USE IEEE.STD_LOGIC_UNSIGNED.ALL; entity clock_enable_debouncing_button is port( clk: in std_logic; -- input clock on FPGA 100Mhz
-- Change counter threshold accordingly slow_clk_enable: out std_logic ); end clock_enable_debouncing_button; architecture Behavioral of clock_enable_debouncing_button is signal counter: std_logic_vector(27 downto 0):=(others => '0'); begin process(clk) begin if(rising_edge(clk)) then counter <= counter + x"0000001"; if(counter>=x"003D08F") then -- reduce this number for simulation counter <= (others => '0'); end if; end if; end process; slow_clk_enable <= '1' when counter=x"003D08F" else '0'; -- reduce this number for simulation
end Behavioral; --fpga4student.com: FPGA projects, Verilog projects, VHDL projects -- VHDL project: VHDL code for debouncing buttons on FPGA -- VHDL D-flip-flop with clock enable signal for debouncing buttons library IEEE; use IEEE.STD_LOGIC_1164.ALL; entity DFF_Debouncing_Button is port( clk: in std_logic; clock_enable: in std_logic; D: in std_logic; Q: out std_logic:='0' ); end DFF_Debouncing_Button; architecture Behavioral of DFF_Debouncing_Button is begin process(clk) begin if(rising_edge(clk)) then if(clock_enable='1') then Q <= D; end if; end if; end process; end Behavioral; --fpga4student.com: FPGA projects, Verilog projects, VHDL projects -- VHDL project: VHDL code for debouncing buttons on FPGA -- VHDL code for button debouncing on FPGA library IEEE; use IEEE.STD_LOGIC_1164.ALL; entity Debouncing_Button_VHDL is port( button: in std_logic; clk: in std_logic; debounced_button: out std_logic ); end Debouncing_Button_VHDL; architecture Behavioral of Debouncing_Button_VHDL is signal slow_clk_enable: std_logic; signal Q1,Q2,Q2_bar,Q0: std_logic; begin clock_enable_generator: entity work.clock_enable_debouncing_button PORT MAP ( clk => clk, slow_clk_enable => slow_clk_enable );
Debouncing_FF0: entity work.DFF_Debouncing_Button PORT MAP ( clk => clk, clock_enable => slow_clk_enable, D => button, Q => Q0 );Debouncing_FF1: entity work.DFF_Debouncing_Button PORT MAP ( clk => clk, clock_enable => slow_clk_enable, D => Q0, Q => Q1 ); Debouncing_FF2: entity work.DFF_Debouncing_Button PORT MAP ( clk => clk, clock_enable => slow_clk_enable, D => Q1, Q => Q2 ); Q2_bar <= not Q2; debounced_button <= Q1 and Q2_bar; end Behavioral;
Testbench VHDL code for debouncing buttons on FPGA:
LIBRARY ieee; USE ieee.std_logic_1164.ALL; --fpga4student.com: FPGA projects, Verilog projects, VHDL projects -- VHDL code for button debouncing on FPGA -- Testbench VHDL code for button debouncing ENTITY tb_debouncing_button_VHDL IS END tb_debouncing_button_VHDL; ARCHITECTURE behavior OF tb_debouncing_button_VHDL IS -- Component Declaration for VHDL code for button debouncing COMPONENT Debouncing_Button_VHDL PORT( button : IN std_logic; clk : IN std_logic; debounced_button : OUT std_logic ); END COMPONENT; signal button : std_logic := '0'; signal clk : std_logic := '0'; signal debounced_button : std_logic; constant clk_period : time := 10 ns; BEGIN -- Instantiate VHDL code for button debouncing uut: Debouncing_Button_VHDL PORT MAP ( button => button, clk => clk, debounced_button => debounced_button ); clk_process :process begin clk <= '0'; wait for clk_period; clk <= '1'; wait for clk_period; end process; -- Stimulus process stim_proc: process begin -- hold reset state for 100 ns. wait for 100 ns; wait for clk_period*10; button <= '0'; wait for 10 ns; button <= '1'; wait for 20 ns; button <= '0'; wait for 10 ns; button <= '1'; wait for 30 ns; button <= '0'; wait for 10 ns; button <= '1'; wait for 40 ns; button <= '0'; wait for 10 ns; button <= '1'; wait for 30 ns; button <= '0'; wait for 10 ns; button <= '1'; wait for 1000 ns; button <= '0'; wait for 10 ns; button <= '1'; wait for 20 ns; button <= '0'; wait for 10 ns; button <= '1'; wait for 30 ns; button <= '0'; wait; end process; END;
Simulation Waveform for the VHDL code:
Only a single pulse is created as shown in the simulation waveform above when the input button is pressed, held long enough, and released.
Recommended VHDL projects:
1. What is an FPGA? How VHDL works on FPGA
2. VHDL code for FIFO memory
3. VHDL code for FIR Filter
4. VHDL code for 8-bit Microcontroller
5. VHDL code for Matrix Multiplication
6. VHDL code for Switch Tail Ring Counter
7. VHDL code for digital alarm clock on FPGA
8. VHDL code for 8-bit Comparator
9. How to load a text file into FPGA using VHDL
10. VHDL code for D Flip Flop
11. VHDL code for Full Adder
12. PWM Generator in VHDL with Variable Duty Cycle
13. VHDL code for ALU
14. VHDL code for counters with testbench
15. VHDL code for 16-bit ALU
16. Shifter Design in VHDL
17. Non-linear Lookup Table Implementation in VHDL
18. Cryptographic Coprocessor Design in VHDL
1. What is an FPGA? How VHDL works on FPGA
2. VHDL code for FIFO memory
3. VHDL code for FIR Filter
4. VHDL code for 8-bit Microcontroller
5. VHDL code for Matrix Multiplication
6. VHDL code for Switch Tail Ring Counter
7. VHDL code for digital alarm clock on FPGA
8. VHDL code for 8-bit Comparator
9. How to load a text file into FPGA using VHDL
10. VHDL code for D Flip Flop
11. VHDL code for Full Adder
12. PWM Generator in VHDL with Variable Duty Cycle
13. VHDL code for ALU
14. VHDL code for counters with testbench
15. VHDL code for 16-bit ALU
16. Shifter Design in VHDL
17. Non-linear Lookup Table Implementation in VHDL
18. Cryptographic Coprocessor Design in VHDL
21. How to generate a clock enable signal instead of creating another clock domain
22. VHDL code for debouncing buttons on FPGA
23. VHDL code for Traffic light controller
24. VHDL code for a simple 2-bit comparator
25. VHDL code for a single-port RAM
26. VHDL code for Car Parking System using FSM
27. VHDL coding vs Software Programming
22. VHDL code for debouncing buttons on FPGA
23. VHDL code for Traffic light controller
24. VHDL code for a simple 2-bit comparator
25. VHDL code for a single-port RAM
26. VHDL code for Car Parking System using FSM
27. VHDL coding vs Software Programming
No comments:
Post a Comment