In this project, a MIPS Assembly program is written to move a virtual point robot on a given environment.
The dimensions of the environment, starting coordinates of the robot, locations of obstacles and moving commands will be provided. The program should move the robot according to the given provided commands, environment dimensions, and obstacle coordinates. At the end of the program, the final coordinate of the robot should be presented.
Below is an example of the input :
3x5#(1,2)#(0,4),(2,3)#ACCAGGGACEEEFFDB#
3x5 is the dimension of the provided environment.
(1,2) is the initial coordinate of the robot.
(0,4) and (2,3) are the coordinates of the obstacles.
# is the character which separates the input parts.
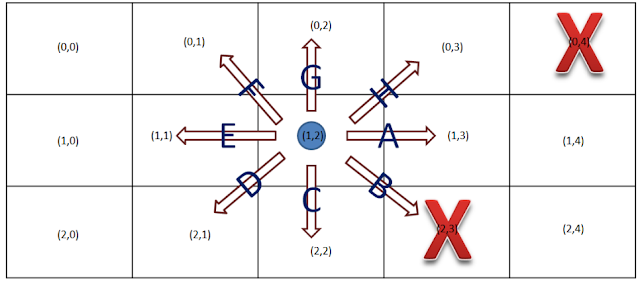
Virtual presentation of the example environment
As we can see in the virtual presentation of the environment, the directions of moving commands (A, B, C, D, E, F, G, and H) are shown.
The expected final coordinate of the robot is (2,2).
When the program receives a moving command, it will check if there is any obstacle at the next position. If it detects an obstacle at the next position, the program should ignore the moving command. Otherwise, the program will move the robot with respect to the moving command.
Below is the MIPS assembly code for the virtual point robot:
############################################ ####### A virtual point robot ############## ############################################ .data input: .space 201 # save the logic expression size_envir: .space 201 # save the dimensions of environment start_coordinate: .space 201 # save the start_coordinate of robot obstacles: .space 201 # save all coordinates of obstacles result: .asciiz "(" sign1: .asciiz "," sign2: .asciiz ")" .text #enables text input / output, kind of like String.h in C++ ######################################### ############### Main ################### ######################################### main: la $a0,input #input for logic expression to get dimensions of environment, starting coordinates... li $a1,201 li $v0,8 syscall # get the logic expression from the console la $a1,size_envir # save the dimensions of environment xori $s0,$a0,0x00 xori $s1,$a1,0x00 lb $t1, 0($s0) sb $t1,0($s1) lb $t1, 2($s0) sb $t1,1($s1) la $a1,start_coordinate # save the starting coordinates of robot xori $s0,$a0,0x00 xori $s1,$a1,0x00 lb $t1, 5($s0) sb $t1,0($s1) lb $t1, 7($s0) sb $t1,1($s1) lb $t6,0($s1) # save x-coordinate of robot lb $t7,1($s1) # save y-coordinate of robot la $a1,obstacles # save the coordinates of obstacles xori $s0,$a0,0x00 xori $s1,$a1,0x00 lb $t1, 11($s0) sb $t1,0($s1) lb $t1, 13($s0) sb $t1,1($s1) addi $a0,$a0,9 addi $a1,$a1,1 check_obstables: # check next obstacles addi $a0,$a0,6 xori $s0,$a0,0x00 lb $t1,0($s0) addi $t2,$zero,35 # detect the "#" character to know whether or not there is no next obstacles. addi $t3,$zero,44 # detect the "," character xori $t4,$s0,0x00 beq $t1,$t2,move_command # perform move commands beq $t1,$t3,next_obstacles # check next obstacles li $v0,10 # code for exit syscall # exit program ######################################################## next_obstacles: addi $t4,$t4,2 xori $s0,$t4,0 lb $t1,0($s0) addi $a1,$a1,1 xori $s1,$a1,0x00 sb $t1,0($s1) addi $a1,$a1,1 xori $s1,$a1,0x00 addi $s0,$s0,2 lb $t1,0($s0) sb $t1,0($s1) j check_obstables ###################################################### move_command: la $a3,obstacles subu $t5,$a1,$a3 #addi $t5,$t5,1 # $t5 is the number of obstacles ####################################################### move_doing: # do the move commands addi $a0,$a0,1 # next move command xori $s0,$a0,0x00 lb $t1,0($s0) addi $t2,$zero,35 beq $t1,$t2,finish # if the command is #, finish the program. otherwise, move on the command ##################################################### # Continue to do the command ##################################################### xori $t2,$zero,0x41 # "A" command beq $t1,$t2,A_command xori $t2,$zero,0x42 # "B" command beq $t1,$t2,B_command xori $t2,$zero,0x43 # "C" command beq $t1,$t2,C_command xori $t2,$zero,0x44 # "D" command beq $t1,$t2,D_command xori $t2,$zero,0x45 # "E" command beq $t1,$t2,E_command xori $t2,$zero,0x46 # "F" command beq $t1,$t2,F_command xori $t2,$zero,0x47 # "G" command beq $t1,$t2,G_command xori $t2,$zero,0x48 # "H" command beq $t1,$t2,H_command li $v0,10 # code for exit syscall # exit program A_command: xori $t1,$t6,0x00 xori $t2,$t7,0x00 addi $t2,$t2,1 addi $t4,$t5,0 j check_border B_command: xori $t1,$t6,0x00 xori $t2,$t7,0x00 addi $t2,$t2,1 addi $t1,$t1,1 addi $t4,$t5,0 j check_border C_command: xori $t1,$t6,0x00 xori $t2,$t7,0x00 addi $t1,$t1,0x01 addi $t4,$t5,0 j check_border D_command: xori $t1,$t6,0x00 xori $t2,$t7,0x00 addi $t1,$t1,0x01 xori $t3,$zero,0x01 sub $t2,$t2,$t3 addi $t4,$t5,0 j check_border E_command: xori $t1,$t6,0x00 xori $t2,$t7,0x00 xori $t3,$zero,0x01 sub $t2,$t2,$t3 addi $t4,$t5,0 j check_border F_command: xori $t1,$t6,0x00 xori $t2,$t7,0x00 xori $t3,$zero,0x01 sub $t2,$t2,$t3 sub $t1,$t1,$t3 addi $t4,$t5,0 j check_border G_command: xori $t1,$t6,0x00 xori $t2,$t7,0x00 xori $t3,$zero,0x01 sub $t1,$t1,$t3 addi $t4,$t5,0 j check_border H_command: xori $t1,$t6,0x00 xori $t2,$t7,0x00 xori $t3,$zero,0x01 sub $t1,$t1,$t3 addi $t2,$t2,1 addi $t4,$t5,0 j check_border check: #t4 is the number of obstacles #t1: x new #t2: y new la $a3,obstacles add $s0,$a3,$t4 lb $t3,0($s0) beq $t3,$t2,keep_1 xori $t3,$zero,0x01 sub $t4,$t4,$t3 j next_check keep_1: xori $t3,$zero,0x01 sub $t4,$t4,$t3 la $a3,obstacles add $s0,$a3,$t4 lb $t3,0($s0) beq $t3,$t1,keep_position j next_check next_check: xori $t3,$zero,0x01 sub $t4,$t4,$t3 bltz $t4,update_robot j check ######################################### check_border: xori $t3,$zero,48 blt $t1,$t3,keep_position xori $t3,$zero,48 blt $t2,$t3,keep_position la $a3, size_envir xori $s0,$a3,0x00 lb $t3,0($s0) beq $t1,$t3,keep_position xori $s0,$a3,0x00 lb $t3,1($s0) beq $t2,$t3,keep_position j check ######################################### update_robot: addi $t6,$t1,0 # x-coordinate addi $t7,$t2,0 # y-coordinate j move_doing ######################################### keep_position: j move_doing ######################################### finish: # finish all the command and output the final coordinate of robot li $v0, 4 # $system call code for print_str la $a0, result # $address of string to print syscall # print the string li $v0, 1 # $system call code for print_int xori $t1,$zero,48 subu $t6,$t6,$t1 xori $a0,$t6,0x00 # $integer to print syscall # print it li $v0, 4 # $system call code for print_str la $a0, sign1 # $address of string to print syscall li $v0, 1 # $system call code for print_int xori $t1,$zero,48 subu $t7,$t7,$t1 xori $a0,$t7,0x00 # $integer to print syscall li $v0, 4 # $system call code for print_str la $a0, sign2 # $address of string to print syscall li $v0,10 # code for exit syscall # exit program ##########################################
The program is verified on Qtspim and got the correct final coordinate of the robot as shown in the following figure.
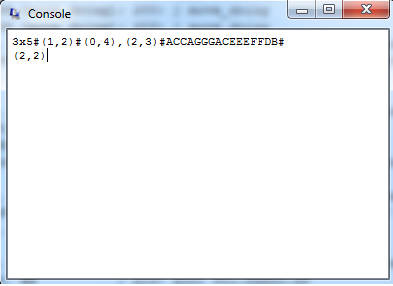
Result of the program
You may like this:
You may also like this:
VHDL code for D Flip FlopVerilog code for D Flip Flop
Verilog code for a comparator
Verilog code for FIFO memory
VHDL code for FIFO memory
Verilog code for 16-bit single-cycle MIPS microprocessor
Programmable digital delay timer in Verilog
Basic digital logic components in Verilog HDL
FIR Filter in VHDL
What is an FPGA? Why FPGA?
A complete 8-bit Microcontroller in VHDL
Verilog code for 32-bit unsigned Divider
Fix-point matrix multiplication in Verilog[Full code and tutorials]
Verilog code for a Carry Look Ahead Multiplier
Programmable digital delay timer in Verilog
Basic digital logic components in Verilog HDL
FIR Filter in VHDL
What is an FPGA? Why FPGA?
A complete 8-bit Microcontroller in VHDL
Verilog code for 32-bit unsigned Divider
Fix-point matrix multiplication in Verilog[Full code and tutorials]
Verilog code for a Carry Look Ahead Multiplier
No comments:
Post a Comment