This FPGA project is aimed to show in details how to process an image using Verilog from reading an input bitmap image (.bmp) in Verilog, processing and writing the processed result to an output bitmap image in Verilog. The full Verilog code for reading image, image processing, and writing image is provided.

In this FPGA Verilog project, some simple processing operations are implemented in Verilog such as inversion, brightness control and threshold operations. The image processing operation is selected by a "parameter.v" file and then, the processed image data are written to a bitmap image output.bmp for verification purposes. The image reading Verilog code operates as a Verilog model of an image sensor/ camera, which can be really helpful for functional verifications in real-time FPGA image processing projects. The image writing part is also extremely useful for testing as well when you want to see the output image in BMP format. In this project, I added some simple image processing code into the reading part to make an example of image processing, but you can easily remove it to get raw image data. All the related questions asked by students are answered at the bottom of this article.
First of all, Verilog cannot read images directly. To read the .bmp image on in Verilog, the image is required to be converted from the bitmap format to the hexadecimal format. Below is a Matlab example code to convert a bitmap image to a .hex file. The input image size is 768x512 and the image .hex file includes R, G, B data of the bitmap image.
First of all, Verilog cannot read images directly. To read the .bmp image on in Verilog, the image is required to be converted from the bitmap format to the hexadecimal format. Below is a Matlab example code to convert a bitmap image to a .hex file. The input image size is 768x512 and the image .hex file includes R, G, B data of the bitmap image.
b=imread('kodim24.bmp'); % 24-bit BMP image RGB888 k=1; for i=512:-1:1 % image is written from the last row to the first row for j=1:768 a(k)=b(i,j,1); a(k+1)=b(i,j,2); a(k+2)=b(i,j,3); k=k+3; end end fid = fopen('kodim24.hex', 'wt'); fprintf(fid, '%x\n', a); disp('Text file write done');disp(' '); fclose(fid); % fpga4student.com FPGA projects, Verilog projects, VHDL projects
To read the image hexadecimal data file, Verilog uses this command: $readmemh or $readmemb if the image data is in a binary text file. After reading the image .hex file, the RGB image data are saved into memory and read out for processing.
Below is the Verilog code to the image reading and processing part:
/******************************************************************************/ /****************** Module for reading and processing image **************/ /******************************************************************************/ `include "parameter.v" // Include definition file // fpga4student.com: FPGA projects for students
// FPGA project: Image processing in Verilog module image_read #( parameter WIDTH = 768, // Image width HEIGHT = 512, // Image height INFILE = "./img/kodim01.hex", // image file START_UP_DELAY = 100, // Delay during start up time HSYNC_DELAY = 160,// Delay between HSYNC pulses VALUE= 100, // value for Brightness operation THRESHOLD= 90, // Threshold value for Threshold operation SIGN=1 // Sign value using for brightness operation // SIGN = 0: Brightness subtraction
// SIGN = 1: Brightness addition ) ( input HCLK, // clock input HRESETn, // Reset (active low) output VSYNC, // Vertical synchronous pulse // This signal is often a way to indicate that one entire image is transmitted. // Just create and is not used, will be used once a video or many images are transmitted. output reg HSYNC, // Horizontal synchronous pulse // An HSYNC indicates that one line of the image is transmitted. // Used to be a horizontal synchronous signals for writing bmp file. output reg [7:0] DATA_R0, // 8 bit Red data (even) output reg [7:0] DATA_G0, // 8 bit Green data (even) output reg [7:0] DATA_B0, // 8 bit Blue data (even) output reg [7:0] DATA_R1, // 8 bit Red data (odd) output reg [7:0] DATA_G1, // 8 bit Green data (odd) output reg [7:0] DATA_B1, // 8 bit Blue data (odd) // Process and transmit 2 pixels in parallel to make the process faster, you can modify to transmit 1 pixels or more if needed output ctrl_done // Done flag ); //------------------------------------------------- // Internal Signals //------------------------------------------------- parameter sizeOfWidth = 8; // data width parameter sizeOfLengthReal = 1179648; // image data : 1179648 bytes: 512 * 768 *3 // local parameters for FSM localparam ST_IDLE = 2'b00,// idle state ST_VSYNC = 2'b01,// state for creating vsync ST_HSYNC = 2'b10,// state for creating hsync ST_DATA = 2'b11;// state for data processing reg [1:0] cstate, // current state nstate; // next state reg start; // start signal: trigger Finite state machine beginning to operate reg HRESETn_d; // delayed reset signal: use to create start signal reg ctrl_vsync_run; // control signal for vsync counter reg [8:0] ctrl_vsync_cnt; // counter for vsync reg ctrl_hsync_run; // control signal for hsync counter reg [8:0] ctrl_hsync_cnt; // counter for hsync reg ctrl_data_run; // control signal for data processing reg [7 : 0] total_memory [0 : sizeOfLengthReal-1];// memory to store 8-bit data image // temporary memory to save image data : size will be WIDTH*HEIGHT*3 integer temp_BMP [0 : WIDTH*HEIGHT*3 - 1]; integer org_R [0 : WIDTH*HEIGHT - 1]; // temporary storage for R component integer org_G [0 : WIDTH*HEIGHT - 1]; // temporary storage for G component integer org_B [0 : WIDTH*HEIGHT - 1]; // temporary storage for B component // counting variables integer i, j; // temporary signals for calculation: details in the paper. integer tempR0,tempR1,tempG0,tempG1,tempB0,tempB1; // temporary variables in contrast and brightness operation integer value,value1,value2,value4;// temporary variables in invert and threshold operation reg [ 9:0] row; // row index of the image reg [10:0] col; // column index of the image reg [18:0] data_count; // data counting for entire pixels of the image //-------------------------------------------------// // -------- Reading data from input file ----------// //-------------------------------------------------// initial begin $readmemh(INFILE,total_memory,0,sizeOfLengthReal-1); // read file from INFILE end // use 3 intermediate signals RGB to save image data always@(start) begin if(start == 1'b1) begin for(i=0; i<WIDTH*HEIGHT*3 ; i=i+1) begin temp_BMP[i] = total_memory[i+0][7:0]; end for(i=0; i<HEIGHT; i=i+1) begin for(j=0; j<WIDTH; j=j+1) begin
// Matlab code writes image from the last row to the first row
// Verilog code does the same in reading to correctly save image pixels into 3 separate RGB mem org_R[WIDTH*i+j] = temp_BMP[WIDTH*3*(HEIGHT-i-1)+3*j+0]; // save Red component org_G[WIDTH*i+j] = temp_BMP[WIDTH*3*(HEIGHT-i-1)+3*j+1];// save Green component org_B[WIDTH*i+j] = temp_BMP[WIDTH*3*(HEIGHT-i-1)+3*j+2];// save Blue component end end end end //----------------------------------------------------// // ---Begin to read image file once reset was high ---// // ---by creating a starting pulse (start)------------// //----------------------------------------------------// always@(posedge HCLK, negedge HRESETn) begin if(!HRESETn) begin start <= 0; HRESETn_d <= 0; end else begin // ______ HRESETn_d <= HRESETn; // | | if(HRESETn == 1'b1 && HRESETn_d == 1'b0) // __0___| 1 |___0____ : starting pulse start <= 1'b1; else start <= 1'b0; end end //-----------------------------------------------------------------------------------------------// // Finite state machine for reading RGB888 data from memory and creating hsync and vsync pulses --// //-----------------------------------------------------------------------------------------------// always@(posedge HCLK, negedge HRESETn) begin if(~HRESETn) begin cstate <= ST_IDLE; end else begin cstate <= nstate; // update next state end end //-----------------------------------------// //--------- State Transition --------------// //-----------------------------------------// // IDLE . VSYNC . HSYNC . DATA always @(*) begin case(cstate) ST_IDLE: begin if(start) nstate = ST_VSYNC; else nstate = ST_IDLE; end ST_VSYNC: begin if(ctrl_vsync_cnt == START_UP_DELAY) nstate = ST_HSYNC; else nstate = ST_VSYNC; end ST_HSYNC: begin if(ctrl_hsync_cnt == HSYNC_DELAY) nstate = ST_DATA; else nstate = ST_HSYNC; end ST_DATA: begin if(ctrl_done) nstate = ST_IDLE; else begin if(col == WIDTH - 2) nstate = ST_HSYNC; else nstate = ST_DATA; end end endcase end // ------------------------------------------------------------------- // // --- counting for time period of vsync, hsync, data processing ---- // // ------------------------------------------------------------------- // always @(*) begin ctrl_vsync_run = 0; ctrl_hsync_run = 0; ctrl_data_run = 0; case(cstate) ST_VSYNC: begin ctrl_vsync_run = 1; end // trigger counting for vsync ST_HSYNC: begin ctrl_hsync_run = 1; end // trigger counting for hsync ST_DATA: begin ctrl_data_run = 1; end // trigger counting for data processing endcase end // counters for vsync, hsync always@(posedge HCLK, negedge HRESETn) begin if(~HRESETn) begin ctrl_vsync_cnt <= 0; ctrl_hsync_cnt <= 0; end else begin if(ctrl_vsync_run) ctrl_vsync_cnt <= ctrl_vsync_cnt + 1; // counting for vsync else ctrl_vsync_cnt <= 0; if(ctrl_hsync_run) ctrl_hsync_cnt <= ctrl_hsync_cnt + 1; // counting for hsync else ctrl_hsync_cnt <= 0; end end // counting column and row index for reading memory always@(posedge HCLK, negedge HRESETn) begin if(~HRESETn) begin row <= 0; col <= 0; end else begin if(ctrl_data_run) begin if(col == WIDTH - 2) begin row <= row + 1; end if(col == WIDTH - 2) col <= 0; else col <= col + 2; // reading 2 pixels in parallel end end end //-------------------------------------------------// //----------------Data counting---------- ---------// //-------------------------------------------------// always@(posedge HCLK, negedge HRESETn) begin if(~HRESETn) begin data_count <= 0; end else begin if(ctrl_data_run) data_count <= data_count + 1; end end assign VSYNC = ctrl_vsync_run; assign ctrl_done = (data_count == 196607)? 1'b1: 1'b0; // done flag //-------------------------------------------------// //------------- Image processing ---------------// //-------------------------------------------------// always @(*) begin HSYNC = 1'b0; DATA_R0 = 0; DATA_G0 = 0; DATA_B0 = 0; DATA_R1 = 0; DATA_G1 = 0; DATA_B1 = 0; if(ctrl_data_run) begin HSYNC = 1'b1; `ifdef BRIGHTNESS_OPERATION /**************************************/ /* BRIGHTNESS ADDITION OPERATION */ /**************************************/ if(SIGN == 1) begin // R0 tempR0 = org_R[WIDTH * row + col ] + VALUE; if (tempR0 > 255) DATA_R0 = 255; else DATA_R0 = org_R[WIDTH * row + col ] + VALUE; // R1 tempR1 = org_R[WIDTH * row + col+1 ] + VALUE; if (tempR1 > 255) DATA_R1 = 255; else DATA_R1 = org_R[WIDTH * row + col+1 ] + VALUE; // G0 tempG0 = org_G[WIDTH * row + col ] + VALUE; if (tempG0 > 255) DATA_G0 = 255; else DATA_G0 = org_G[WIDTH * row + col ] + VALUE; tempG1 = org_G[WIDTH * row + col+1 ] + VALUE; if (tempG1 > 255) DATA_G1 = 255; else DATA_G1 = org_G[WIDTH * row + col+1 ] + VALUE; // B tempB0 = org_B[WIDTH * row + col ] + VALUE; if (tempB0 > 255) DATA_B0 = 255; else DATA_B0 = org_B[WIDTH * row + col ] + VALUE; tempB1 = org_B[WIDTH * row + col+1 ] + VALUE; if (tempB1 > 255) DATA_B1 = 255; else DATA_B1 = org_B[WIDTH * row + col+1 ] + VALUE; end else begin /**************************************/ /* BRIGHTNESS SUBTRACTION OPERATION */ /**************************************/ // R0 tempR0 = org_R[WIDTH * row + col ] - VALUE; if (tempR0 < 0) DATA_R0 = 0; else DATA_R0 = org_R[WIDTH * row + col ] - VALUE; // R1 tempR1 = org_R[WIDTH * row + col+1 ] - VALUE; if (tempR1 < 0) DATA_R1 = 0; else DATA_R1 = org_R[WIDTH * row + col+1 ] - VALUE; // G0 tempG0 = org_G[WIDTH * row + col ] - VALUE; if (tempG0 < 0) DATA_G0 = 0; else DATA_G0 = org_G[WIDTH * row + col ] - VALUE; tempG1 = org_G[WIDTH * row + col+1 ] - VALUE; if (tempG1 < 0) DATA_G1 = 0; else DATA_G1 = org_G[WIDTH * row + col+1 ] - VALUE; // B tempB0 = org_B[WIDTH * row + col ] - VALUE; if (tempB0 < 0) DATA_B0 = 0; else DATA_B0 = org_B[WIDTH * row + col ] - VALUE; tempB1 = org_B[WIDTH * row + col+1 ] - VALUE; if (tempB1 < 0) DATA_B1 = 0; else DATA_B1 = org_B[WIDTH * row + col+1 ] - VALUE; end `endif /**************************************/ /* INVERT_OPERATION */ /**************************************/ `ifdef INVERT_OPERATION value2 = (org_B[WIDTH * row + col ] + org_R[WIDTH * row + col ] +org_G[WIDTH * row + col ])/3; DATA_R0=255-value2; DATA_G0=255-value2; DATA_B0=255-value2; value4 = (org_B[WIDTH * row + col+1 ] + org_R[WIDTH * row + col+1 ] +org_G[WIDTH * row + col+1 ])/3; DATA_R1=255-value4; DATA_G1=255-value4; DATA_B1=255-value4; `endif /**************************************/ /********THRESHOLD OPERATION *********/ /**************************************/ `ifdef THRESHOLD_OPERATION value = (org_R[WIDTH * row + col ]+org_G[WIDTH * row + col ]+org_B[WIDTH * row + col ])/3; if(value > THRESHOLD) begin DATA_R0=255; DATA_G0=255; DATA_B0=255; end else begin DATA_R0=0; DATA_G0=0; DATA_B0=0; end value1 = (org_R[WIDTH * row + col+1 ]+org_G[WIDTH * row + col+1 ]+org_B[WIDTH * row + col+1 ])/3; if(value1 > THRESHOLD) begin DATA_R1=255; DATA_G1=255; DATA_B1=255; end else begin DATA_R1=0; DATA_G1=0; DATA_B1=0; end `endif end end endmodule
The image processing operation is selected in the following "parameter.v" file. To change the processing operation, just switch the comment line.
/***************************************/ /****************** Definition file ********/ /************** **********************************************/ `define INPUTFILENAME "your_image.hex" // Input file name `define OUTPUTFILENAME "output.bmp" // Output file name // Choose the operation of code by delete // in the beginning of the selected line //`define BRIGHTNESS_OPERATION `define INVERT_OPERATION //`define THRESHOLD_OPERATION // fpga4student.com FPGA projects, Verilog projects, VHDL projects
The following Verilog code is to write the processed image data to a bitmap image for verification:
/****************** Module for writing .bmp image *************/ /***********************************************************/ // fpga4student.com FPGA projects, Verilog projects, VHDL projects // Verilog project: Image processing in Verilog module image_write #(parameter WIDTH = 768, // Image width HEIGHT = 512, // Image height INFILE = "output.bmp", // Output image BMP_HEADER_NUM = 54 // Header for bmp image ) ( input HCLK, // Clock input HRESETn, // Reset active low input hsync, // Hsync pulse input [7:0] DATA_WRITE_R0, // Red 8-bit data (odd) input [7:0] DATA_WRITE_G0, // Green 8-bit data (odd) input [7:0] DATA_WRITE_B0, // Blue 8-bit data (odd) input [7:0] DATA_WRITE_R1, // Red 8-bit data (even) input [7:0] DATA_WRITE_G1, // Green 8-bit data (even) input [7:0] DATA_WRITE_B1, // Blue 8-bit data (even) output reg Write_Done ); // fpga4student.com FPGA projects, Verilog projects, VHDL projects //-----------------------------------// //-------Header data for bmp image-----// //-------------------------------------// // Windows BMP files begin with a 54-byte header initial begin BMP_header[ 0] = 66;BMP_header[28] =24; BMP_header[ 1] = 77;BMP_header[29] = 0; BMP_header[ 2] = 54;BMP_header[30] = 0; BMP_header[ 3] = 0;BMP_header[31] = 0; BMP_header[ 4] = 18;BMP_header[32] = 0; BMP_header[ 5] = 0;BMP_header[33] = 0; BMP_header[ 6] = 0;BMP_header[34] = 0; BMP_header[ 7] = 0;BMP_header[35] = 0; BMP_header[ 8] = 0;BMP_header[36] = 0; BMP_header[ 9] = 0;BMP_header[37] = 0; BMP_header[10] = 54;BMP_header[38] = 0; BMP_header[11] = 0;BMP_header[39] = 0; BMP_header[12] = 0;BMP_header[40] = 0; BMP_header[13] = 0;BMP_header[41] = 0; BMP_header[14] = 40;BMP_header[42] = 0; BMP_header[15] = 0;BMP_header[43] = 0; BMP_header[16] = 0;BMP_header[44] = 0; BMP_header[17] = 0;BMP_header[45] = 0; BMP_header[18] = 0;BMP_header[46] = 0; BMP_header[19] = 3;BMP_header[47] = 0; BMP_header[20] = 0;BMP_header[48] = 0; BMP_header[21] = 0;BMP_header[49] = 0; BMP_header[22] = 0;BMP_header[50] = 0; BMP_header[23] = 2;BMP_header[51] = 0; BMP_header[24] = 0;BMP_header[52] = 0; BMP_header[25] = 0;BMP_header[53] = 0; BMP_header[26] = 1; BMP_header[27] = 0; end //---------------------------------------------------------// //--------------Write .bmp file ----------------------// //----------------------------------------------------------// initial begin fd = $fopen(INFILE, "wb+"); end always@(Write_Done) begin // once the processing was done, bmp image will be created if(Write_Done == 1'b1) begin for(i=0; i<BMP_HEADER_NUM; i=i+1) begin $fwrite(fd, "%c", BMP_header[i][7:0]); // write the header end for(i=0; i<WIDTH*HEIGHT*3; i=i+6) begin // write R0B0G0 and R1B1G1 (6 bytes) in a loop $fwrite(fd, "%c", out_BMP[i ][7:0]); $fwrite(fd, "%c", out_BMP[i+1][7:0]); $fwrite(fd, "%c", out_BMP[i+2][7:0]); $fwrite(fd, "%c", out_BMP[i+3][7:0]); $fwrite(fd, "%c", out_BMP[i+4][7:0]); $fwrite(fd, "%c", out_BMP[i+5][7:0]); end end end
The header data for the bitmap image is very important and it is published here. If there is no header data, the written image could not be correctly displayed. In Verilog HDL, $fwrite command is used to write data to file.
Next, let's write a test bench Verilog code to verify the image processing operations.
`timescale 1ns/1ps /**************************************************/ /******* Testbench for simulation ****************/ /*********************************************/ // fpga4student.com FPGA projects, Verilog projects, VHDL projects // Verilog project: Image processing in Verilog `include "parameter.v" // include definition file module tb_simulation; //------------------ // Internal Signals //------------------------------------------------- reg HCLK, HRESETn; wire vsync; wire hsync; wire [ 7 : 0] data_R0; wire [ 7 : 0] data_G0; wire [ 7 : 0] data_B0; wire [ 7 : 0] data_R1; wire [ 7 : 0] data_G1; wire [ 7 : 0] data_B1; wire enc_done; image_read #(.INFILE(`INPUTFILENAME)) u_image_read ( .HCLK (HCLK ), .HRESETn (HRESETn ), .VSYNC (vsync ), .HSYNC (hsync ), .DATA_R0 (data_R0 ), .DATA_G0 (data_G0 ), .DATA_B0 (data_B0 ), .DATA_R1 (data_R1 ), .DATA_G1 (data_G1 ), .DATA_B1 (data_B1 ), .ctrl_done (enc_done) ); image_write #(.INFILE(`OUTPUTFILENAME)) u_image_write ( .HCLK(HCLK), .HRESETn(HRESETn), .hsync(hsync), .DATA_WRITE_R0(data_R0), .DATA_WRITE_G0(data_G0), .DATA_WRITE_B0(data_B0), .DATA_WRITE_R1(data_R1), .DATA_WRITE_G1(data_G1), .DATA_WRITE_B1(data_B1), .Write_Done() ); //------------- // Test Vectors //------------------------------------- initial begin HCLK = 0; forever #10 HCLK = ~HCLK; end initial begin HRESETn = 0; #25 HRESETn = 1; end endmodule
Finally, we have everything to run a simulation to verify the image processing code. Let's use the following image as the input bitmap file:

Input bitmap image
Run the simulation for 6ms, close the simulation and open the output image for checking the result. Followings are the output images which are processed by the selected operations in parameter.v:
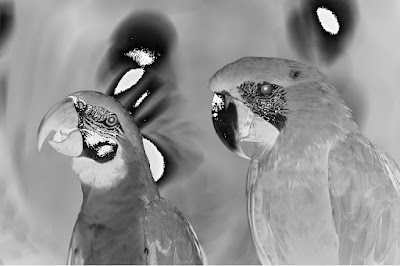
Output bitmap image after inverting

Output bitmap image after threshold operation

Output bitmap image after subtracting brightness
Since the reading code is to model an image sensor/camera for simulation purposes, it is recommended not to synthesize the code. If you really want to synthesize the processing code and run this directly on FPGA, you need to replace these image arrays (total_memory, temp_BMP, org_R, org_B, org_G) in the code by block memory (RAMs) and design address generators to read image data from the block memory.
After receiving so many questions related to this project, followings are the answers to your questions:
1. Explanation and tutorial on how to run the simulation:
2. The full Verilog code for this image processing project can be downloaded here. Run the simulation about 6ms and close the simulation, then you will be able to see the output image.
3. The reading part operates as a Verilog model of an image sensor/camera (output RGB data, HSYNC, VSYNC, HCLK). The Verilog image reading code is extremely useful for functional verification in real-time FPGA image/video projects.
4. In this project, I added the image processing part to make an example of image enhancement. You can easily remove the processing part to get only raw image data in the case that you want to use the image sensor model only for verifying your image processing design.
5. The image saving into three separate RGB mems: Because Matlab code writes the image hexadecimal file from the last row to the first row, the RGB saving codes (org_R, org_B, org_G) do the same in reading the temp_BMP memory to save RGB data correctly. You can change it accordingly if you want to do it differently.
6. You might find the following explanation for the BMP header useful if you want to change the image size:
Image size = 768*512*3= 1179648 bytes
BMP header = 54 bytes
BMP File size = Image size + BMP Header = 1179702 Bytes
Convert it to hexadecimal numbers: 1179702 in Decimal = 120036 in Hexadecimal
Then 4-byte size of BMP file: 00H, 12 in Hexa = 18 Decimal, 00H, 36 in Hexa = 54 Decimal
That's how we get the following values:
BMP_header[ 2] = 54;
BMP_header[ 3] = 0 ;
BMP_header[ 4] = 18;
BMP_header[ 5] = 0 ;
Image width = 768 => In hexadecimal: 0x0300. The 4 bytes of the image width are 0, 3, 0, 0. That's how you get the following values:
BMP_header[18] = 0;
BMP_header[19] = 3;
BMP_header[20] = 0;
BMP_header[21] = 0;
Image height = 512 => In hexadecimal: 0x0200. The 4 bytes of the image width are 0, 2, 0, 0. That's how we get the following values:
BMP_header[22] = 0;
BMP_header[23] = 2;
BMP_header[24] = 0;
BMP_header[25] = 0;
7. You should not synthesize this code because it is not designed for running on FPGA, but rather for functional verification purposes. If you really want to synthesize this code (read and process) and load the image into FPGA for processing directly on FPGA, replace all the temp. variables (org_R, org_B, org_G, tmp_BMP = total_memory) by block RAMs and generate addresses to read the image data (remove the always @(start) and all the "for loops" - these are for simulation purposes). There are two ways: 1. write a RAM code and initialize the image data into the memory using $readmemh; 2. generate a block memory using either Xilinx Core Generator or Altera MegaFunction and load the image data into the initial values of memory (.coe file for Xilinx Core Gen. and .mif for Altera MegaFunction), then read the image data from memory and process it (FSM Design).
8. In this project, two even and old pixels are read at the same time for speeding up the processing, but you can change the number of pixels being read depending on your design.
9. The writing Verilog code is also very helpful for testing purposes as you can see the output in BMP format.
10. If you want to do real-time image processing, you can check this for the camera interface code: Basys 3 FPGA OV7670 Camera
29. Verilog code for Multiplexers
3. The reading part operates as a Verilog model of an image sensor/camera (output RGB data, HSYNC, VSYNC, HCLK). The Verilog image reading code is extremely useful for functional verification in real-time FPGA image/video projects.
4. In this project, I added the image processing part to make an example of image enhancement. You can easily remove the processing part to get only raw image data in the case that you want to use the image sensor model only for verifying your image processing design.
5. The image saving into three separate RGB mems: Because Matlab code writes the image hexadecimal file from the last row to the first row, the RGB saving codes (org_R, org_B, org_G) do the same in reading the temp_BMP memory to save RGB data correctly. You can change it accordingly if you want to do it differently.
6. You might find the following explanation for the BMP header useful if you want to change the image size:
Image size = 768*512*3= 1179648 bytes
BMP header = 54 bytes
BMP File size = Image size + BMP Header = 1179702 Bytes
Convert it to hexadecimal numbers: 1179702 in Decimal = 120036 in Hexadecimal
Then 4-byte size of BMP file: 00H, 12 in Hexa = 18 Decimal, 00H, 36 in Hexa = 54 Decimal
That's how we get the following values:
BMP_header[ 2] = 54;
BMP_header[ 3] = 0 ;
BMP_header[ 4] = 18;
BMP_header[ 5] = 0 ;
Image width = 768 => In hexadecimal: 0x0300. The 4 bytes of the image width are 0, 3, 0, 0. That's how you get the following values:
BMP_header[18] = 0;
BMP_header[19] = 3;
BMP_header[20] = 0;
BMP_header[21] = 0;
Image height = 512 => In hexadecimal: 0x0200. The 4 bytes of the image width are 0, 2, 0, 0. That's how we get the following values:
BMP_header[22] = 0;
BMP_header[23] = 2;
BMP_header[24] = 0;
BMP_header[25] = 0;
7. You should not synthesize this code because it is not designed for running on FPGA, but rather for functional verification purposes. If you really want to synthesize this code (read and process) and load the image into FPGA for processing directly on FPGA, replace all the temp. variables (org_R, org_B, org_G, tmp_BMP = total_memory) by block RAMs and generate addresses to read the image data (remove the always @(start) and all the "for loops" - these are for simulation purposes). There are two ways: 1. write a RAM code and initialize the image data into the memory using $readmemh; 2. generate a block memory using either Xilinx Core Generator or Altera MegaFunction and load the image data into the initial values of memory (.coe file for Xilinx Core Gen. and .mif for Altera MegaFunction), then read the image data from memory and process it (FSM Design).
8. In this project, two even and old pixels are read at the same time for speeding up the processing, but you can change the number of pixels being read depending on your design.
9. The writing Verilog code is also very helpful for testing purposes as you can see the output in BMP format.
10. If you want to do real-time image processing, you can check this for the camera interface code: Basys 3 FPGA OV7670 Camera
Recommended Verilog projects:
2. Verilog code for FIFO memory
3. Verilog code for 16-bit single-cycle MIPS processor
4. Programmable Digital Delay Timer in Verilog HDL
5. Verilog code for basic logic components in digital circuits
6. Verilog code for 32-bit Unsigned Divider
7. Verilog code for Fixed-Point Matrix Multiplication
8. Plate License Recognition in Verilog HDL
9. Verilog code for Carry-Look-Ahead Multiplier
10. Verilog code for a Microcontroller
11. Verilog code for 4x4 Multiplier
12. Verilog code for Car Parking System
13. Image processing on FPGA using Verilog HDL
14. How to load a text file into FPGA using Verilog HDL
15. Verilog code for Traffic Light Controller
16. Verilog code for Alarm Clock on FPGA
17. Verilog code for comparator design
18. Verilog code for D Flip Flop
19. Verilog code for Full Adder
20. Verilog code for counter with testbench
21. Verilog code for 16-bit RISC Processor
22. Verilog code for button debouncing on FPGA
23. How to write Verilog Testbench for bidirectional/ inout ports
3. Verilog code for 16-bit single-cycle MIPS processor
4. Programmable Digital Delay Timer in Verilog HDL
5. Verilog code for basic logic components in digital circuits
6. Verilog code for 32-bit Unsigned Divider
7. Verilog code for Fixed-Point Matrix Multiplication
8. Plate License Recognition in Verilog HDL
9. Verilog code for Carry-Look-Ahead Multiplier
10. Verilog code for a Microcontroller
11. Verilog code for 4x4 Multiplier
12. Verilog code for Car Parking System
13. Image processing on FPGA using Verilog HDL
14. How to load a text file into FPGA using Verilog HDL
15. Verilog code for Traffic Light Controller
16. Verilog code for Alarm Clock on FPGA
17. Verilog code for comparator design
18. Verilog code for D Flip Flop
19. Verilog code for Full Adder
20. Verilog code for counter with testbench
21. Verilog code for 16-bit RISC Processor
22. Verilog code for button debouncing on FPGA
23. How to write Verilog Testbench for bidirectional/ inout ports
24. Tic Tac Toe Game in Verilog and LogiSim
25. 32-bit 5-stage Pipelined MIPS Processor in Verilog (Part-1)
26. 32-bit 5-stage Pipelined MIPS Processor in Verilog (Part-2)
27. 32-bit 5-stage Pipelined MIPS Processor in Verilog (Part-3)
28. Verilog code for Decoder25. 32-bit 5-stage Pipelined MIPS Processor in Verilog (Part-1)
26. 32-bit 5-stage Pipelined MIPS Processor in Verilog (Part-2)
27. 32-bit 5-stage Pipelined MIPS Processor in Verilog (Part-3)
29. Verilog code for Multiplexers
30. N-bit Adder Design in Verilog
31. Verilog vs VHDL: Explain by Examples
32. Verilog code for Clock divider on FPGA
33. How to generate a clock enable signal in Verilog
34. Verilog code for PWM Generator
35. Verilog coding vs Software Programming
36. Verilog code for Moore FSM Sequence Detector
37. Verilog code for 7-segment display controller on Basys 3 FPGA
31. Verilog vs VHDL: Explain by Examples
32. Verilog code for Clock divider on FPGA
33. How to generate a clock enable signal in Verilog
34. Verilog code for PWM Generator
35. Verilog coding vs Software Programming
36. Verilog code for Moore FSM Sequence Detector
37. Verilog code for 7-segment display controller on Basys 3 FPGA
Hi Van,
ReplyDeleteMy name is Jewel. I'm from India doing B.tech in Manipal University Your code on image processing using verilog HDL was really helpful. I'm doing a project called JPEG encoder using verilog HDL for grayscale image. Can u please help me with a code for this topic?
Regards,
JEWEL DOMINIC SAVIO ANTONY
Email me please. Thanks.
DeleteVan!
Deleteif u have a code related to JPEG compression using forward discrete cosine transform then please send me,,my email id is hamzaali776819@gmail.com
may i get full image processing vhdl
Deleteplease share the code to akhilparakkat@gmail.com
Deletebaydarbasar@yahoo.com
ReplyDeleteEmailed you. Kindly keep up to date with FPGA projects using Verilog/ VHDL fpga4student.com. Thanks
DeleteHello Van Loi Le, code is very useful. Thanks. Can you please send me full verilog source code and test bench , with the image you have used here
Deletethanks in advance
(revanthaero@gmail.com)
You are welcome. Please help to like and share the site with your friends: https://www.facebook.com/fpga4student and keep updates with coming projects.Thanks.
ReplyDeleteHello Van Loi Le, code is very useful. Thanks. Can you please send me full verilog source code
ReplyDeleteKindly keep up to date with FPGA projects using Verilog/ VHDL fpga4student.com. Thanks
DeleteWhat is your email?
ReplyDeleteHello please share project my email: sylaslly@gmail.com
ReplyDeleteEmailed you. Kindly keep up to date with FPGA projects using Verilog/ VHDL fpga4student.com. Thanks
Deletehello can you share the project to me too? honhonteriteri@gmail.com
DeleteHi code is very useful please send me full code
ReplyDeletemy mail id is niranjanmehar445@gmail.com
ReplyDeleteEmailed you. Kindly keep up to date with FPGA projects using Verilog/ VHDL fpga4student.com. Thanks
Deletehey niranjanmehar445@gmail.com
Deletecan you send me the code
Email: nishantsingla07@gmail.com
Can you please send me the full verilog source files? My mail id is gitalive@gmail.com. It'd be very useful for my project in image processing.
ReplyDeletePleeach check your email
ReplyDeleteHi van, can you provide me full source code , I want to learn this project , thx a lot
Deletemy email : dance84327@gmail.com
Can you please sned the full verilog source files. My email is cmonterrosad@gmail.com.
ReplyDeleteKindly check your email.
DeleteHi,your code is very useful for me. please share project my email: adabi.1373@yahoo.com
ReplyDeletethank you.
Emailed you. Kindly keep up to date with FPGA projects using Verilog/ VHDL fpga4student.com. Thanks
DeleteHi,can you please share me the code freely since i can not pay for that. ill be so helpful if you are kind to do this.
Deletehi...I find your code more useful in my project. . Can you please share me. . My email: annette307@gmail.com
ReplyDeleteHi,
ReplyDeleteKindly check your email
Excellent Implementation.
DeleteCan you please forward me the entire verilog file so that I can use in my project,
Email: akash.nomul0310@gmail.com
I have a doubt regarding the two variables used "total_memory" and "sizeOfLengthReal". What values are to be entered?
These are size of the image memory. It depends on your image size.
DeleteHello please share project my email:thinkalot812@gmail.com
ReplyDeleteHi, my email id is : singhadiaashish@gmail.com , may you please email me the source code.
ReplyDeletemail me code :heena09shaikh@gmail.com
ReplyDeletevipul4336@gmail.com,thanks :)
ReplyDeletecould you please email me the code
ReplyDeleteomersalim4901@gmail.com
Exquisite tutorial on image processing on FPGA using Verilog HDL. I thank you for sharing it. Color Correction Service plays a vital role in image manipulation.
ReplyDeletecan u please share this code ? my mail id is swarnah235@gmail.com .....
ReplyDeletethanks in advance.
cool!
ReplyDeletefasma@mailinator.com
Very helpful source. i need to load a video file in fpga. can you help me and procedure what i have to follow? thanks in advance.
ReplyDeletemy mail id is: sivamanik.44@gmail.com
Sir,i have followed your blog since 2016 and currently i am doing a project on verilog to design a codec using wavelet transform algorithm,sir i require your help regarding this.Can you help me in completing my project?
ReplyDeletenice post ! genwen.zhao@gmail.com
ReplyDeletevery good!! i would like to use your code. email anurung@gmail.com
ReplyDeleteCan you please send me the code @ sabarishprasanna@gmail.com
ReplyDeleteCan you mail the code to harshagopal1@gmail.com ?
ReplyDeleteHello, I would greatly appreciate if you could share your code to darkinthewind@live.com
ReplyDeleteThank you!
please send us the full code and do we need zed-board for the processing the output? my email address is radha.rani9924@gmail.com. Thanks in advance...
ReplyDeleteBravo sir. you have done a great job it will assist the new learners like me a lot. Sir can you plz share the code with me so i can practice it. sir please share the full code with me at my email address nasirkhanpak25@gmail.com
ReplyDeleteThanks in advance. Wish you best for luck.
Kindly check your email.
ReplyDeleteAdmin
I need the complete fpga code.. can I have so?
ReplyDeleteWhat is your email?
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteCould you please send me the source code. My email address is thelight.bn@gmail.com. Thank you in advance!
ReplyDeletecan ı get the full verilog code of this process to use in my project, please? It will be so helpful for me. mail: eren199461@gmail.com
ReplyDeleteCould you send me the full verilog code please, it's would be very helpful for my project. By the way as a new learner it will help me a lot thank you very much. My email: boy-zaza-@hotmail.com.
ReplyDeleteCould you send me the full verilog code please, my email: messhajar@gmail.com and thanks alot
ReplyDeletecould you please send me full verilog code?
ReplyDeleteemail: paria_gol@ymail.com
thank you
could you please send me full verilog code, it's would be very helpful for my project.
ReplyDeleteemail: srinuaruva97@gmail.com
thank you
It's very useful for me. Could you send me the full verilog code please?
ReplyDeleteMy email: okgreentea@gmail.com
Hello,
ReplyDeleteThis is amazing can i ask for full verilog code? I'm doing one personal project and full code would help me a lot thanks.
Email: frosty.ever@gmail.com
hey frosty.ever@gmail.com
Deletecan you send me the code
Email: nishantsingla07@gmail.com
Please check your email
ReplyDeleteplease mail me too ..
Deleteplease send it to me. My email is kabujop4life@gmail.com. Thanks
ReplyDeleteplease send me the codes. My email id is abhishek16961@gmail.com
ReplyDeleteLOI LE VAN ... Please mail me the full code as soon as possible it's urgent ... thank you
ReplyDeletemy mail id is gupta.shri16@gmail.com
please mail me the full code. My email is vinayak.r30@gmail.com
Deletethanks.
can ı get the full verilog code of this process to use in my project. It will be so helpful for me. mail: malliksomu1@gmail.com
ReplyDeleteSomeone has the code? can you send me?
ReplyDeletefabyes95@gmail.com
can you plz send me full code at mayuruttarwar98@gmail.com
ReplyDeleteCan you send me the code, calebhillary97@gmail.com Thank You
ReplyDeleteVery good work sir , I would love to have a look at the full code .
ReplyDeletePlease email it to me at sai00201@gmail.com
Great work sir, Can you send me the code, calebhillary97@gmail.com Thank You
ReplyDeleteGreat project thanks
ReplyDeleteCan you send me the whole code , thanks in advance , cekinmezabdulkerim@gmail.com
ReplyDeleteSeems very useful Loi , appreciate it if you send the full source code , mehmetmert.bese@gmail.com
ReplyDeleteplz send full source code to meetjana007@gmail.com
ReplyDeletecan I ask you?
ReplyDeleteI can't use image processing by using verilog. So how to input with image and take output with image?
Which language are you using?
ReplyDeleteverilog hdl.
DeleteThe challenge is to process the output from the vedio driver to fpga.Since I'm a beginner, I can't even see the input and output.
please send me a file. boxisfun@gmail.com
ReplyDeletePlease make it clear, The post showed how to read and write images.
ReplyDeletethanks, but the code make me help. so please.
Deletethanks, but the code make me help. so please.
Deleteprat.abhay@gmail.com
ReplyDeleteKindly Mail
Hello, thank you for information, could you send me the source code cagriyalcin01@gmail.com thank you
ReplyDeleteThanks, that's great. But on what board did you implement these code?
ReplyDeletePleas send me complete code, my email id is mdanishamir@gmail.com
ReplyDeleteHello, thank you for information, could you send me the source code sureshcme15@gmail.com thank you
ReplyDeleteCan you please send the entire code
ReplyDeleteMy email id-ashisharya30@gmail.com
Thank You...
hello this code is very useful.
ReplyDeleteplease send to code
gmlwjd6135@naver.com
Hello van.
ReplyDeleteCan you please send me the full verilog source files?
It will be very useful to me.
thank you. have a nice day.
my email is jcy9476@naver.com
Hello Van,
ReplyDeletecould you send me the vhdl code? Thanks
minosse89@hotmail.it
Hello, thank you for information, could you also send me the source code kennethibarra.7416@gmail.com thank you
ReplyDeleteCan I get the source code please? honhonteriteri@gmail.com
ReplyDeletehello can anyone give me full code for this project my email is kmp8072@gmail.com
ReplyDeletehello can anyone give me full code for this project my email is alibaran78@hotmail.com
ReplyDeleteHi Lợi, can you send me full code for this project? I have a project about image processing using Machine Learning and I do not know read a file to a buffer and transfer data from a buffer to another buffer. My email is lehongtuandinh@gmail.com. Thanh you very much for your help!!!
ReplyDeletePlease send me the full code sir.I want to do this as my project. Thanks in advance.
ReplyDeleteligtruth@gmail.com
pokemonygz@gmail.com
ReplyDeletecan u send me code
no
DeleteHello could you send me the source code please ? atakancelikkol57@gmail.com
ReplyDeleteCan I get the source code please? chenkai525@gmail.com
ReplyDeleteCan I get the source code please? chenkai525@gmail.com
ReplyDeleteCheck your emails. Thanks.
ReplyDeletehello sir, please could yo e-mail me the full code (juanrome93@gmail.com) i'm trying to do a project in which i need to load an image in a fpga to do some processing to it, it would very helpfully to have you full code.
ReplyDeletecould you please email me the source code at myblckmajic2@gmail.com
ReplyDeleteCould you please kindly email me the source code at myblackmajic2@gmail.com . I provided an incorrect email id the previous time.
ReplyDeletehi...I find your code more useful in my project. . Can you please share me. . My email: sarojshrestha4411@gmail.com
ReplyDeletehi,,,
ReplyDeleteif u have a verilog hdl code of jpeg image compression than please send me,,,
my email id is hamzaali776819@gmail.com
Great!!!
ReplyDeletePlease email me. nganhnam_1307@yahoo.com
good informations.
ReplyDeletecan i get your full source code please?
my e-mail adrress is qq11123@naver.com
good informations.
ReplyDeletecan i get your full source code please?
my e-mail adrress is qq11123@naver.com
I'm unable to execute the code which you given because i didn't understand what are the inputs and outputs clearly and how the memory is allocated. Can you please help me...
ReplyDeleteSir, I'm unable to execute the code because I did not understand that what are the total memory and SizeOflengthReal-1. I'm doing a project on that.So can you send me the code please...?
ReplyDeletemy g-mail address is:sandhyavasanthapanduri@gmail.com
The provided code is a good start in image processing on FPGA. Spend some time to figure it out.
ReplyDeleteTotal memory is the memory to read the image data from .hex file.
SizeOflengthReal-1 is the size of the total memory. Image size is 768x512 pixels. Each pixel has 3 data (R-G-B). Each datum R or G or B has 8 bits. Then you can calculate the total memory size.
Your example is exactly as I need. Can you email the source code to johnbeer@att.net Thank you.
ReplyDeleteThe provided code is good enough for you to start an image processing project on FPGA. Spend some time to figure it out.
ReplyDeletewhat should be the i and j values..
ReplyDeleteHi, Thanks. I updated the i and j code values. Basically, i and j are the index of the memory to read from or write into all the pixels(R,G,B) of the image.
Deleteeducative post . Liked and appreciate it . keep continue sharing .
ReplyDeleteThanks for your kind words. Greatly appreciate it. Sure will continue to give more educative FPGA projects.
DeleteThank you, this post is very educative and explained in good manner.please mail me full source code. my mail id is pk.smvdu@gmail.com .
ReplyDeleteCould you send me the source code please ? dynamogen64@gmail.com
ReplyDeleteCould anyone explain how he computed file size in byte (the 4 bytes after 2 signature bytes 'BM' in BITMAP header) please? I'm very confuse. I tried to convert to hexadecimal then compute the file size but it look like not true.
ReplyDeleteBMP_header[ 2] = 54;
BMP_header[ 3] = 0 ;
BMP_header[ 4] = 18;
BMP_header[ 5] = 0 ;
Image size = 768*512*3= 1179648 bytes
DeleteBMP header = 54 bytes
BMP File size = Image size + BMP Header = 1179702 Bytes
Convert it to hexadecimal numbers: 1179702 in Decimal = 120036 in Hexadecimal
Then 4-byte size of BMP file: 00H, 12 in Hexa = 18 Decimal, 00H, 36 in Hexa = 54 Decimal
That's how you get the values:
BMP_header[ 2] = 54;
BMP_header[ 3] = 0 ;
BMP_header[ 4] = 18;
BMP_header[ 5] = 0 ;
this is a very nice project....is it possible for me to get the full source codes??
ReplyDeleteif so please can someone send them to me at mohanty.sunny7@gmail.com
Could you please send the source code to navya.doe@gmail.com?
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteHi all,
ReplyDeleteThe provided information on this post are pretty enough for you to get started with image processing on FPGA. Please spend some time to figure it out so that you can learn a lot from the experience.
This post is a tutorial for image processing on FPGA. Full code will not be provided.
Thanks.
Admin
If you have any question, you can ask me via admin@fpga4student.com or comment here. I will help to answer all of the questions.
ReplyDelete# ERROR: C:/Modeltech_5.5e/examples/verilog1.v(2): near "#": expecting: ';'
ReplyDelete# ERROR: C:/Modeltech_5.5e/examples/verilog1.v(49): near "[": expecting: ';'
# ERROR: C:/Modeltech_5.5e/examples/verilog1.v(55): near "]":syntax error
# ERROR: C:/Modeltech_5.5e/examples/verilog1.v(56): near "]":syntax error
I am getting these errors when i compile the module for reading and processing.
This post is a tutorial for image processing on FPGA. Full code is not given, but the major part for reading and writing image were provided. Please spend some time to figure it out so that you can learn a lot from the experience.
DeleteYes,but i am not able to undertsand where the syntax error is ,even in write module it shows same errors,like near "[": expecting: ';',as above errors,i don't know how to correct it.
DeleteThere is also error for parameter in read as well as write module,near "#": expecting: ';'
You need to add more code to make it works. Spend some time to figure it out.
DeleteThis code very useful! Could you please send full code to me? My email is nguyenhaitan0907@gmail.com
ReplyDeleteThank you very much!
This code is very useful. Please send it to me. My email is nguyenhaitan0907@gmail.com
ReplyDeleteThank you!
can you send me the full verilog code.
ReplyDeletenirod_s@yahoo.com
Hey,
ReplyDeleteThe site is very helpful, can you send me the full verilog code to my email.
nirod_s@yahoo.com
Can you please send the full verilog code
ReplyDeleteMy email is vinayak.r30@gmail.com
Thanks
can someone send the full verilog code. My email is vinayak.r30@gmail.com
ReplyDeletethanks.
my name is tin. I am a student and am studying the filter canny implemented on the FPGA. ADmin has a project on canny filter? help me .
ReplyDeletecan you send me?
tintin1134@gmail.com
thank you
Hi, your code is very useful .
ReplyDeleteCan you please send me full code .
Thank You
Email:- nishantsingla07@gmail.com
can you please send me the full code .
ReplyDeletenishantsingla07@gmail.com
Hi, your code is very useful .
ReplyDeleteCan you please send me full code .
Thank You
Email:- thongvan631@gmail.com
can you please send me full verilog code for ASK on FPGA?
ReplyDeletethank you
email: onelove55563@gmail.com
hey can you send me the full verilog code too for xilinx?
ReplyDeletemy email is naufal.bap@gmail.com
ur help is appreciated thanks
I am learning basics of image processing using fpga
ReplyDeleteCould you please send me the code
It would be of great help
saicharanrachamadugu@ymail.com
kindly send me code at my email nainshah.shah@gmail.com
ReplyDeletehi, I am wondering what "parameter.v" is.
ReplyDeleteAmazing... Thanks for uploading this project online. I am new to FPGAs and trying to learn image processing on FPGA. This project is an ideal to start up with. Could you please share source code with me on prashantborhade36@gmail.com
ReplyDeletecan you please send me the code?
ReplyDeleteCan you send me the full verilog code?
ReplyDeleteMy email: akngndz93@gmail.com
Thank you for your help.
Hi, your code is very useful I need to learn more about this.
ReplyDeleteCan you please send me source code .
Email: thanaphan2008@hotmail.com
Hello, I am a project with Xilinx FPGA.
ReplyDeletecurrently, I can not input a bitmap in my project code.
If you share your full code. my project will upgrade.
Could you send me full code?
My email address is shun435@gmail.com.
Thank you for your help.
Hello, I am a project with Xilinx FPGA.
ReplyDeletecurrently, I can not input a bitmap in my project code.
If you share your full code. my project will upgrade.
Could you send me full code?
My email address is shun435@gmail.com.
Thank you for your help.
Can you please send me source code .Thank you
ReplyDeleteEmail: duyviet210894@gmail.com
Hi I would be happy for the source code, thanks a lot!!
ReplyDeletebarakbattach@gmail.com
Hi I would be happy for the source code, thanks a lot!!
ReplyDeletebarakbattach@gmail.com
Hi, first of all many thanks for putting up these codes. I have used this as a reference material to build a verilog code that could invert a grayscale image. You have here used system verilog functions like $fopen and $fwirte. When I compile the program it states that these system functions are not synthesizable. Did you have the same issue?
ReplyDelete$fopen and $fwrite are not synthesizable. These are for writing image file on PC for verification.
Deletehello! can u please send the source code
Deletetejaswinitm898@gmal.com
can you please send me the full code.
ReplyDeleteosk702@naver.com
can you please send me the code?
ReplyDeleteCan you please share the full Verilog code: hemachandu62@gmail.com
ReplyDeleteCan you please share the complete Verilog code to hemachandu62@gmail.com
ReplyDeleteHello Van,
ReplyDeleteCan you please share the code for full project. It will be a huge help for me.
My email monzurularash1@gmail.com
Thank you
Monzurul
This comment has been removed by the author.
ReplyDeleteKindly can you share me the code for this page:
ReplyDeleteMy Email is hazoor786@gmail.com
Hello..your code seems very useful for my project. Can you please send me the full verilog code. Thank you.. good job
ReplyDeleteemail: rita.abiakl@hotmail.com
Can i have the full coding please ? Because it is a very useful reference for my fyp which is implementation of fractal image compression on FPGA. I can share you my full coding once I get the results. My email is matrices3176@gmail.com
ReplyDeleteThank you . Your help is greatly appreciate.
Hi Van
ReplyDeleteThis is very helpful for me. Could you also consider send me the source code. Thank you very much. My email is: zzmzc333@gmail.com
Sincerely,
Zhiming
Hi Van
ReplyDeleteThis is very helpful for me. Could you also consider send me the source code. Thank you very much. This is my email: zzmzc333@gmail.com
Sincerely,
Zhiming
Can you share this project code with me please it will be a huge help.
ReplyDeleteMy email: am070794@gmail.com
Can u mail it to me too pls ...the full code... Email :arunarena123@gmail.com
ReplyDeleteHi,your code is helpful.
ReplyDeleteCan you send the code to me.
Thank you very much.
My e-mail : alex10130010@gmail.com
This was very helpful. Could you send me the whole code as soon as possible.
ReplyDeleteMy mail ID is manchikalapudi.laxmi.15ece@bml.edu.in
sir can u please share the full code for image processing using verilog hdl
ReplyDeleteemail ID:sanchavihj1997@gmail.com
Hello
ReplyDeletei want to use an image in my laptop as input of testbench.i do not know verilog code for this.my mean is that i give the address of image(address of place that my image saved in my laptop) and use from that image such as input.please help me.thank you
Hi, Can you send me this project with me ? Thanks
ReplyDeletegunduzozk@gmail.com
can u send me complete code of this project
ReplyDeletemy mail id rajputanilkumar@gmail.com
Hi, your code is very useful in my Project . Can you please share the complete Verilog code.
ReplyDeleteEmail : 12ece18@gmail.com
Hello sir, can you send me the source code please? Will be a huge help. Thanks
ReplyDeletemy email: thiagorodrigues.c@gmail.com
Van, i cant synthesis the code as parameter.v is not not found. What can i do, can u send me v files for the project? I am new for image procesing
ReplyDeleteHi van, can you provide me parameter.v file as i am getting the error in that part.
ReplyDeleteDear Admin,
ReplyDeleteI am grateful to this knowledge transfer.
kindly send me the parameter.v file and other supported file for running this simulation.
manimail2007@gmail.com
Dear Admin,
ReplyDeleteCan you please send the entire project files to this email: sakreddy7@gmail.com
Thanks in advance.
Hi everyone, as there are so many requests, I updated the full code for this project.
ReplyDeleteHi,your code is very useful for me. please share project my email: dance84327@gmail.com
ReplyDeletethank you.
Hi Van
ReplyDeleteI have a trouble processing the image
and I can't see the right output result
this is my email:ayn77543@gmail.com
If you see this comment can you send a email to me so that I can tell more details.
Thanks a lot.
If you have any questions, email at admin@fpga4student.com
DeleteCan anyone tell me how to create the HEX file ?
ReplyDeleteRead the tutorial. There is a Matlab code to do that.
DeleteCan i get full Vhdl code for my research ? plz
ReplyDeleteHi, this is a great resource, thanks so much for sharing. I am trying to build a code in Verilog that can read video files and analyze spectral data. It would be very helpful if you share any knowledge on that as well as email the full source code for this. My email: fariahmahzabeen@gmail.com. Will greatly appreciate your help, many thanks!
ReplyDeletepls mail the full code ..my email id is muthuprathiba@gmail.com
ReplyDeletepls mail the full code ..my email id is muthuprathiba@gmail.com
ReplyDeleteHello Van Loi Le, code is very useful. Thanks. Can you please send me full verilog source code and test bench , with the image you have used here
ReplyDeletecan you help in other projects ,i have a project related to image registration using verilog
my email address is: zaidalsadane@gmail.com
Hello van.
ReplyDeleteCan you please send me the full verilog source files?
It will be very useful to me.
thank you. have a nice day.
my email is:zaidalsadane@gmail.com
i also have a project image regitration using verilog codes can you help me
Hi man
ReplyDeleteCan u please send me total verilog code please
My email is kailase999nikhil@gmail.com